Why Do We Lint?
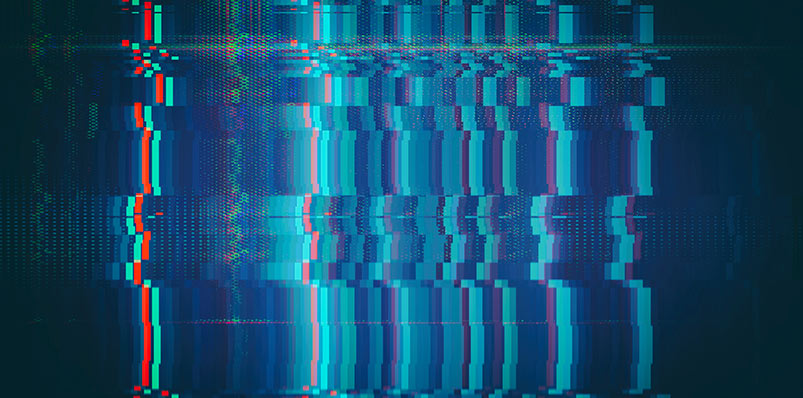
- Linting
- Analyzing code for inconsistent or questionable patterns.
Linting has become one of the more ubiquitous practices across all types of languages and frameworks. But have you wondered why it’s found in so many enterprise, personal, and open source projects? What has driven so much adoption in a field where there are so many differing opinions on what’s necessary?
|
|
Take the function above as an example. Messy, right? There’s a few glaring inconsistencies here - the indentation is all over the place, snake_case is mixed with camelCase and PascalCase, differing forms of comments are used, commas are sprinkled in too liberally or not at all…
Now, bear in mind this is valid code (at least for our example), but reading it can be tricky, and trying to update or add to the code without completely reformatting it would be unsatisfying.
If we point a javascript linter at it (for this example, we’ll use the google eslint standard), we see output like the following:
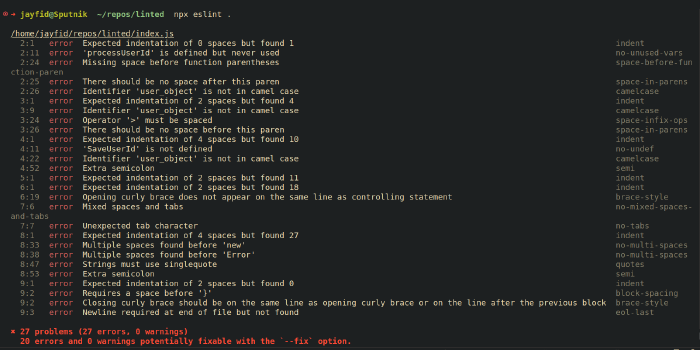
Dozens of linting errors!
That’s a lot of errors. Looks like my nine lines of code are in pretty bad shape!
Running the linter again, this time with the --fix
flag to automatically fix
common issues, the number of remaining issues goes down to just four errors.
After dealing with those by hand, we have something that looks like this:
|
|
This is much more orderly! Putting personal preferences aside for the moment, it’s consistent and you can add to or modify it without worrying about conflicting styles.
You may notice that the two versions, while functionally the same, are written
quite differently. Most notably the cleaned-up version defines an arrow function
in place of the traditional javascript function
, the user_object
argument
has been replaced with
object destructuring,
and the if/else statements have been inlined. This demonstrates the nature of
linting and possibly a limitation of static analyzers - while some stylistic
choices can be restricted by the linter, there are still many style choices that
make it into the code. If we wanted to, we could continue to edit this function
without changing what it does. For example, we could eliminate the else
statement and simply throw if the condition on line 3 isn’t met:
|
|
So now we’ve managed to restate the same functionality in 3 different ways without actually improving or degrading the logic. In terms of whether the style we landed on is definitive, now and in the future, it’s absolutely not. This is simply one of many possibilities within the rules dictated by Google’s current linting standards (as of the writing of this article). Those standards will inevitably evolve over time. Not to mention, there are also competing standards out there. If we point the popular AirBnB linting standard at this latest revision, we’ll see this:
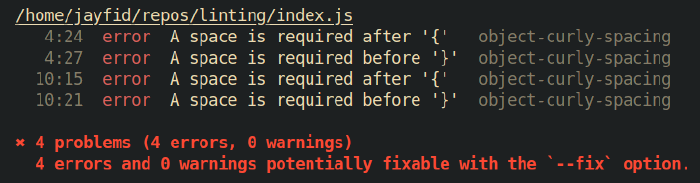
These AirBnB errors conflict with the current Google standard we originally used.
There have always been numerous ways to solve the same problem going back to the earliest days of software engineering. Back in the day, it was extremely common to view files where multiple developers had made contributions using different/conflicting styles. It wasn’t uncommon to see an ungodly mix of tabs and spaces in the same project, the same file, or even the same line. The natural entropy of this environment led to an unbelievable sprawl of stylistic choices. There were artistic uses of whitespace and comments, often meant to illustrate themes that were completely indecipherable to anyone but the original developer. Giant comment blocks attempted to segment code into sections and give files a logical structure. Ascii art was occasionally thrown in to try to tell some kind of story about what you were looking at.
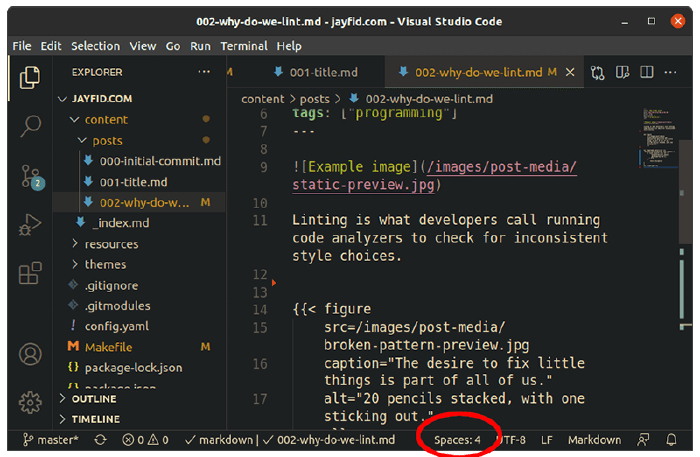
The legacy of the ‘spaces’ vs ’tabs’ war is still present in every code editor. That setting, along with text encoding and line endings, remains the most visible (and least changed) setting in every current developer’s workspace.
Since those days, there have been hundreds of new developments in coding styles. Every major language has tried its hand at creating order out of chaos. And every major language has its own linters. If you wanted, you could dig through thousands of online posts between developers arguing the more pedantic nuances of edge-case style standards. Oftentimes heatedly. Usually sardonically. Occasionally quite caustically.
One big change came in the early 2000s when the Python
language became
immensely popular. In python
, whitespace is part of the syntax. Code needed to
be properly indented or it simply wouldn’t work. There continued to be many,
many other styling issues, but this was the first time most developers got a
glimpse of something not being valid, functioning code just because of how it
looked.
But since the early days, the single largest (IMO) change to utilitarian linting has been its prominent inclusion in frontend build-chains (the tools used to develop and deploy websites). Build chains generally offer things like the ability to preprocess files, view a live preview in your browser, and package everything up for deployment to real servers. Each popular framework also includes a linter for HTML/CSS/JS (as well as their offshoots HTML5/SCSS/LESS/JSX/TYPESCRIPT/etc…). Some build chains’ linting is so deeply embedded and strict that the project will fail to build if a single line looks funky.
Loyalty to one’s build chain became another hot topic for developers. Time and again, new and incompatible paradigms took the frontend community by storm. The tenor of discussion seemed to become a one-upmanship contest, with each voice vying to be more technically correct than the last. Given that environment, it’s no wonder that all of the projects created in the intervening years have such varied and irreparably fractured styles.
But back on topic, let’s answer my opening question: Why do we lint?
For many developers today, that answer is likely “because the build-chain tells me to”. From a purist perspective, linting should be a convenience for teams and OSS - a fast-track to an agreement about a trivial aspect of the solution. But why then is there so much debate? So much quibbling and redefining? Why can’t there be an immutable set of centralized standards that everyone adheres to now and forever? It’s not because there can’t be. If everyone’s preferences were ignored, an arbitrary standard could take hold. Even in the small percentage of cases where linting rules must be ignored to make the project work, there are ways to bypass and ignore the linter.
To any developer reading this, I would ask: do you have a linter installed in your personal and private projects? In the likely case that you do, how often does it run? Would it truly matter if some lines in your blog templates don’t conform to popular standards?
The real answer is this: Developers are compulsively obsessed with order and patterns. I don’t mean this in a medically diagnostic manner. We certainly don’t all suffer from a legitimate disorder. But as a group of people, we enjoy getting into code and molding it like clay. As we work, patterns elucidate themselves in our minds. We don’t just seek to codify lines of computer instructions - we seek to create order that defines the whole project and sits on top of the lines, files, and directories like meta-code. And frankly, it can be quite enjoyable. Linting is mental masturbation. It feels good and has a quick feedback loop for creating order and achieving a goal. It’s a low-stakes way to feel like you’re making progress without achieving anything at all. In the sometimes daunting world of problem-solving, linting is a dopamine hit waiting to be farmed. There’s no ambiguity in following an error message to the exact line you need to fix and then fixing it. And seeing it line up perfectly with every other line can be very gratifying. Did you feel a bit of satisfaction seeing the block of code up top get cleaned up? I know I did.
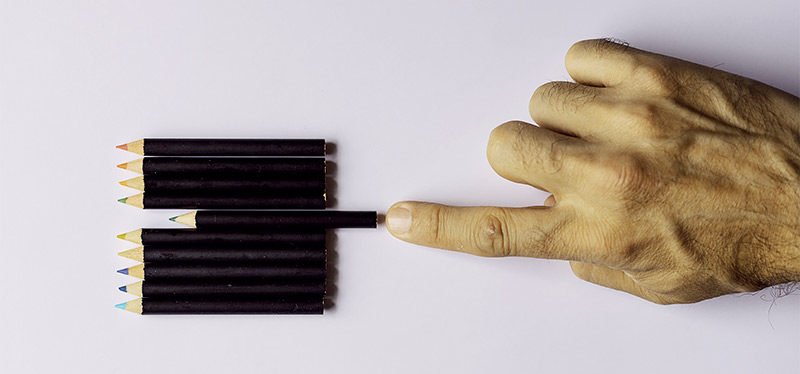
The desire to organize is within all of us.
But I will say - in the world of enterprise software engineering, where many developers need to quickly jump into a repo and grok what’s happening so they can make their own edits, it’s important to practice discipline when the siren song of organizing begins to tempt you. In the best of times, it will lead you down a road of wasted effort with no demonstrable progress being made. In the worst of times, when the drive to create order becomes unbalanced, the end result is a huge mess of meta programming and threads of half-implemented patterns that only the original author could ever hope to untangle. Much like the OCD sufferer who, in their desire to feel clean, washes their hands until they’re dry, cracked, and bloody - the unbounded pursuit of perfect order inevitably leads beyond “good enough” and starts to wear away at the foundation.
So in conclusion, we lint on this massive scale because it scratches an itch. We quibble because our guts tell us that certain forms of order are “best” and alternatives to that are “bad”, but ultimately it’s all a delusion. If you can tell what code is doing and how you could clean it up, then that code is clear enough and shouldn’t be touched. It’s critical in team-based projects that we balance our desire for organization and find alternate outlets for it. I find personal websites/apps/firmware projects to be ideal for this purpose. When I have time, I can sit down and decide to make forward progress, or I can reorganize things so they’re “just right”. Either way, I enjoy the process. Having that outlet, I’m able to approach work where people are paying me to reach a goal in a much more sober manner. While planning upcoming work, I can clearly discern what is valuable work and what’s just “feel good refactoring”.
To me, this realization is a critical step in the maturation of developers - because no one ever tells us that following our gut on what’s “best practice” or an “anti-pattern” can be delusional thinking. Once you realize that reorganizing things to your liking isn’t necessary or valuable, it frees you up to truly hear the opinions of others and to make a real impact.
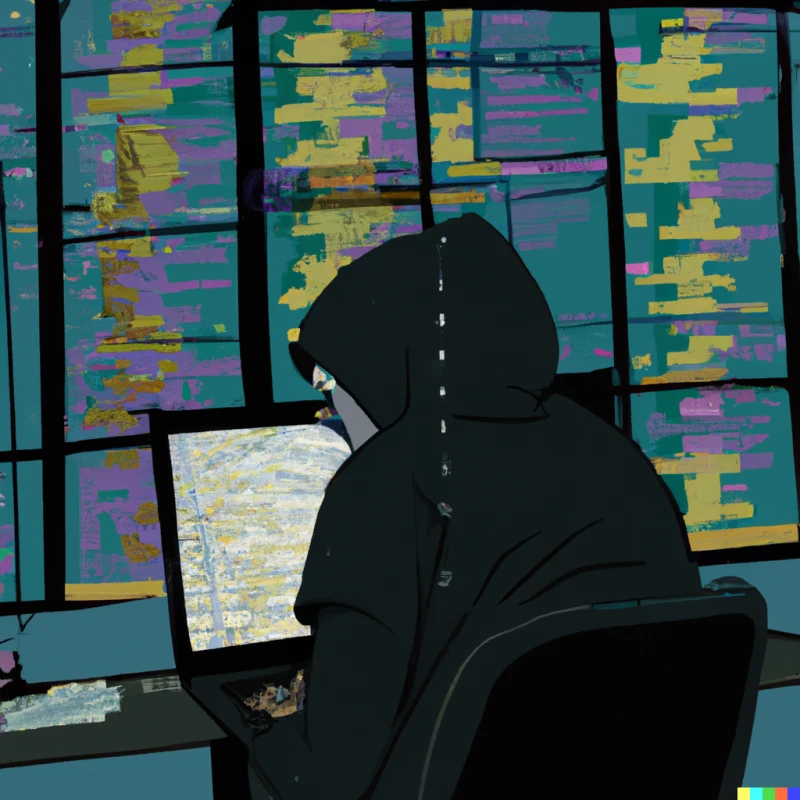
Dall-e generated art from the prompt: Digital art of a programmer desperately organizing their code